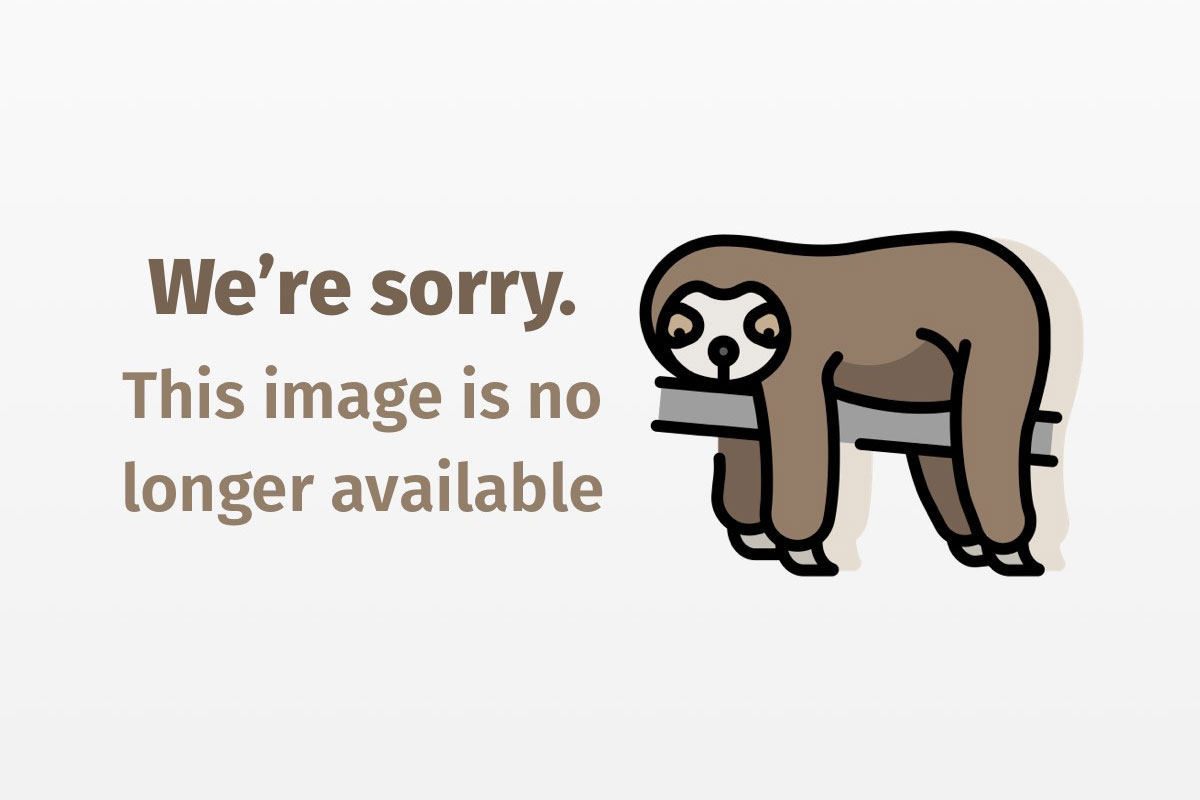
Java Tip 123: Dial into the wireless world
Execute a simple phone book application with WML and JSP
We have all heard the phrase killer wireless application. Unfortunately, those killer apps are more complicated than they look. Competing standards, myriad devices, and different browsers create a difficult development landscape. In this tip, I focus on cell phone development to show how to simplify that landscape. You will use the Wireless Markup Language (WML), a leading language for developing wireless applications, and the Wireless Application Protocol (WAP), a protocol mainly used to deliver content to cell phones. I will cover the basics of creating, deploying, and executing a WAP application using the Apache Tomcat Web server and Nokia Mobile Internet Toolkit.
WAP application architecture
When a user presses a cell phone key to access a Website, a WAP request is made to that cell phone’s registered gateway. The WAP gateway decodes and translates the request into an HTTP request. The gateway routes the request to the Web server serving that request’s URL. The Web server processes the request, and then delivers the HTTP response back to the gateway. The gateway translates the HTTP response into a WAP response and encodes it. It then delivers the response back to the cell phone. Figure 1 illustrates this process.

To understand the WAP application architecture and the proceeding example, you must understand several key components of wireless development: WAP, WML, and the WAP gateway.
WAP
The WAP specification consists of a wireless data protocol. That protocol standardizes the way in which a wireless device’s micro browser communicates with a WAP gateway. The WAP specifications define a set of protocols in application, session, transaction, security, and transport layers that enable operators, manufacturers, and application providers to meet the challenges in advanced wireless-service differentiation and fast/flexible service creation. The specification also includes the Wireless Markup Language (WML). For general information on WAP, see Resources.
WML
The XML-based WML specifies the content and user interface for WAP-enabled devices. WML for WAP applications is analogous to HTML for HTTP applications. A WML document is a collection — referred to as a deck — of one or more cards. Each card is a well-defined unit of interaction between a user agent and a user (such as a menu, a text screen, or a text-entry field). Each card generally carries enough information to fit into one wireless device screen. For general information on WML, see Resources.
WAP gateway
As Figure 1 shows, the WAP gateway acts as the bridge between the wireless network containing wireless clients and the computer network containing application servers. A WAP gateway typically includes the following functionality:
-
Protocol gateway: The protocol gateway translates requests from the WAP protocol stack to the WWW protocol stack (HTTP and TCP/IP).
- Content encoders and decoders: The content encoders translate Web content into compact encoded formats to reduce the number and size of packets traveling over the wireless data network. When a wireless client sends a request to a WAP application running on a Web server, the request first routes through the WAP gateway where it is decoded, translated to HTTP, and then forwarded to the appropriate URL. The response then reroutes back through the gateway, which translates the response to WAP, encodes it, and forwards it to the wireless client.
This proxy architecture allows application developers to build services that are network and terminal independent. For general information on the WAP gateway, see Resources.
The phone book example
The phone book program is quite simple: you just look up a phone number of a person listed in a phone/address book on your server. You will use the Nokia Simulator client application to simulate your cell phone. The screen will present options to the user for locating phone numbers via the Select option. Once the name is selected, the cell phone will display the phone number on the screen. On the server side, you will use the Apache Tomcat server. Figure 2 illustrates this architecture.

The phone book system follows the WAP application architecture quite closely. First, the Nokia simulated WAP client requests the URL (you can download this article’s source code in Resources). The cell phone sends the WAP request through the built-in Nokia WAP server gateway (in real life, the gateway is located somewhere in the wireless network). That gateway generates a conventional HTTP request for the specified URL and sends it to the Tomcat Web server. Tomcat parses the HTTP request and determines what to retrieve.
As usual, if the URL specifies the static file, the Web server retrieves the file and returns it. On the other hand, if the URL is linked with a Java servlet, Common Gateway Interface (CGI), or other script application, the Web server passes the request to an associated application. In the example, you request the static file phonebook.wml
. The phonebook.wml
file is the WML deck file for the phone book example.
The Web server returns the WML deck with the appropriate HTTP headers. The WAP gateway verifies the response HTTP headers and WML content, then encodes them into binary form. From that, the gateway creates a WAP response and sends it back to the simulated cell phone client.
The cell phone client’s micro browser interprets the WML and displays the first deck on the screen. The browser in Figure 3 shows the options for locating phone numbers via the Select button. When the user selects the name of the person whose phone number he or she wants, another WAP request routes through the WAP gateway to the Web server to locate that number.

You can also select all five persons listed under that option, as Figure 4 shows.

Once you have the name, request the following URL to look up the phone number in the phone book application:
<go
href="
name=$(name:escape)"/>
For example, if you select “Kim,” then that name replaces the $(name:escape)
parameter in the URL sent to the server. However, if you select “All,” then the name field remains empty.
The Tomcat server directs this request to PhoneBookSample.jsp
(download source code). That JSP (JavaServer Page) reads a phonelist
file (a text file) that lists names and phone numbers. The JSP compares the name with that list, and then sends a matching phone number back in WML format. If no name is selected, then it returns the entire phone list as a WML table.
Note that the response’s MIME type is the WML response type rather than the usual HTML type, since that is the nature of this application (and WAP phones don’t understand HTML). To set the MIME type, you add the following line to the top of the PhoneBookSample.jsp
file:
<%@ page contentType="text/vnd.wap.wml"%>
Without this line, the MIME type will default to the HTML MIME type. Note that while some WAP gateways may attempt to automatically translate HTML documents into WML, you have no way to know that translation’s results, so it’s wise to explicitly generate the WML yourself.
Gather all the pieces
You will need to install the following software to run this example:
-
Download the free Apache Tomcat 4.0.1 server.
-
Nokia Mobile Internet Toolkit 3.0 provides an easy way to simulate WAP-enabled mobile phones. It will also serve as your WAP gateway. You can download a 30-day free trial of its latest version at the Nokia WAP Developer Forum.
-
Install the Java Runtime Environment (JRE) 1.3.1 (or higher) software. If you don’t already have this installed, the Nokia Toolkit will automatically install it for you.
-
Unzip
Phonebook.zip
intod:PhoneBook
. If you prefer a different directory, then change thePhoneBookSample.jsp
accordingly. - Create a
phonelist
file, liked:Phonebookphonelist
, and fill it with names and numbers.
Run the example
To run the phone book example, first go to the <TOMCAT_HOME>conf
directory, open server.xml
, and where it says "<!-- Tomcat Root Context -->"
, copy the following:
<Context path="/PhoneBook" docBase="D:/PhoneBook" debug="0"
reloadable="true"/>
Then start the Tomcat Web server.
Next, start the Nokia Mobile Toolkit. The toolkit lets you view WML content the same way users of a WAP-compliant mobile phone would view it. From the Start menu, choose Programs->Nokia Mobile Internet Toolkit->Mobile Internet Toolkit. This also starts the internal WAP gateway.
From the toolkit’s Browser menu, select Load Location. In the Open Location dialog box, enter:
Note that the URL is case sensitive.
From the toolkit’s simulation phone handset:
- Select Options
- Scroll to Select name and hit Select
- Scroll to a listed name, hit Select, and then hit OK
- Select Options
- Scroll to Get number, and hit Select
The simulated mobile client will contact PhoneBookSample.jsp
to obtain the phone number and display the results in the handset.
If your Nokia Active Server and Tomcat are running on two different machines, you must modify the phonebook.wml
and replace the local host by the Tomcat server machine’s IP address. If you find an error while running this program, try running directly from the Internet browser by typing the URL you are trying to reach. The Internet browser will give you a more detailed error, so you can easily debug the problem. If you have problems with the Nokia simulator, try closing and relaunching it. Problems are not common and may only occur when a user makes a typing mistake and the page is not reloaded.
The killer app
Now you know how to run a simple wireless application. The software you downloaded for the example contains various examples and beginner guides to get you started. Feel free to replace Tomcat with a more robust Web server, such as BEA WebLogic, Netscape Enterprise Server, or Microsoft IIS, for enterprise-level applications. You’re all set to evolve the techniques presented here to build your own killer wireless apps.