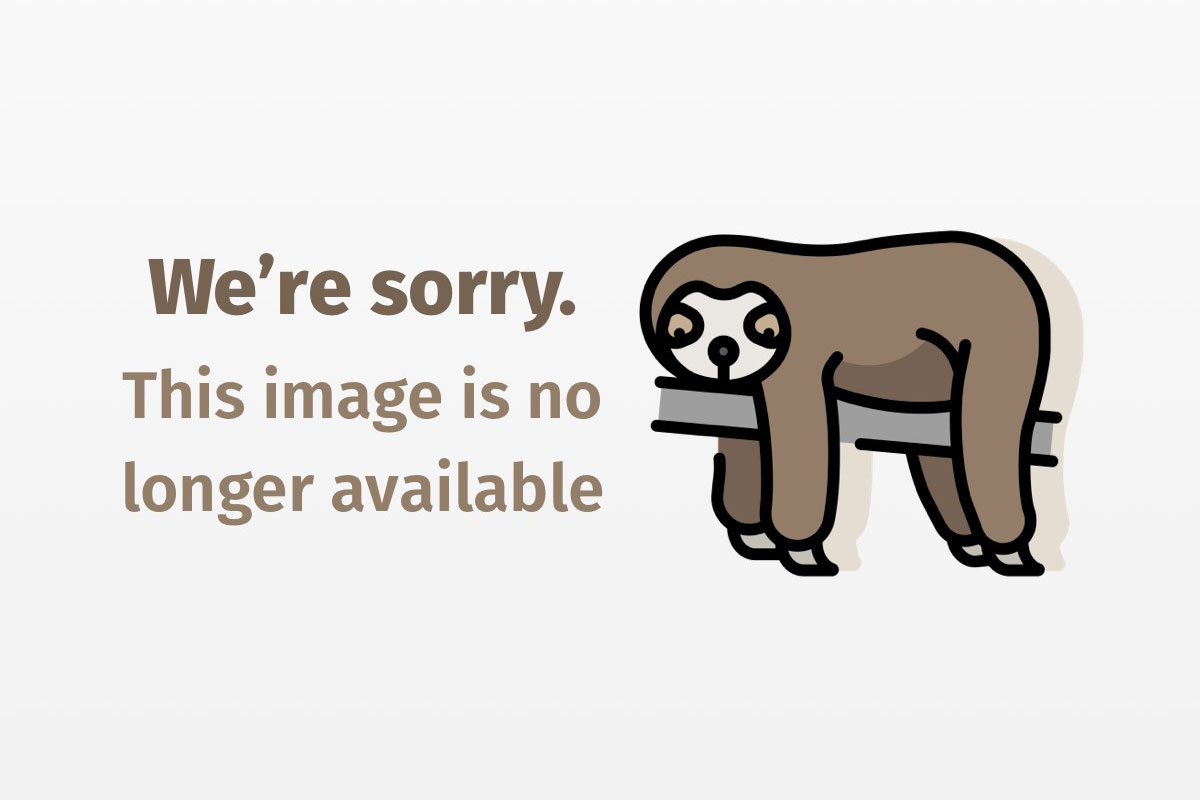
Deliver cellular messages with SMS
SMS: A shortcut to providing information services to cell phones
The mobile communication device market is growing exponentially. A survey by Gartner Dataquest predicted that worldwide mobile phone sales will have totaled 412.7 million units in 2000, a 45.5 percent increase from 1999. (See Resources for a link to the survey.) This growth, coupled with the Internet’s evolution into a Web of services, has fueled demand for Internet-enabled services that wireless devices — particularly cell phones — can readily access. Many technologies can be harnessed to provide value-added services over a cell phone, including Wireless Application Protocol (WAP) and Short Message Service (SMS).
Developed by the WAP Forum, WAP is a standard for providing Internet communications to wireless devices like cell phones, PDAs, and pagers. WAP was expected to be the predominant standard for providing ubiquitous mobile Internet access; however, several reports indicate that WAP is unlikely to succeed in the short- to medium-term. Because of insufficient infrastructural investments in gateways, WAP-enabled handsets did not coevolve to support this service. There are lingering performance concerns as well. In a survey conducted by the department of mass communications at Tamkang University (Taiwan), over 80 percent of WAP-enabled cell phone users expressed dissatisfaction with WAP. (See Resources.) The reasons cited included speed — rather, lack of it — expense, and lack of content. Those factors might lead to a dead-on-arrival situation with WAP, which directly affects application developers. It makes little sense to invest in a technology that might not survive.
SMS is a viable standard that offers a platform for delivering wireless information services. SMS cannot match WAP’s wireless browsing capabilities, but it can meet the demand for point-to-point message-based services. SMS-enabled information services become even more attractive when you compare the large base of installed SMS-enabled cell phones to the paltry number of WAP-ready phones. According to a March 2000 report on internet.com — “Companies Seek to Bridge ‘WAP Gap,’” (see Resources for a link) — over 400 million legacy (non-WAP-enabled) digital wireless phones are in use today.
Banking on SMS’s productivity, we propose a server-side Java-based solution that provides information services to cell phones via SMS and Web form scraping.
What is SMS?
Developed in 1991, SMS is a globally accepted wireless service that enables the transmission of alphanumeric messages between mobile subscribers and external systems like email, paging, and voice mail systems.
With SMS, an active mobile handset can receive or submit a short message at any time, even if a voice or data call is in progress. SMS also guarantees delivery of the short message by the network. Temporary failures due to unavailable receiving stations are identified, and the short message is stored until the destination device becomes available.
Initial applications of SMS focused on eliminating alphanumeric pagers by permitting two-way general-purpose messaging and notification services, primarily for voice mail. As technology and networks evolved, a variety of services were introduced, including interactive banking, information services such as stock quotes, integration with Internet-based applications, and email, fax, and paging integration. In addition, integration with the Internet spurred the development of Web-based messaging and other interactive applications such as instant messaging, gaming, and chatting.
Here are a few reasons why SMS, not WAP, should be used for value-added services in the short- to medium-term:
- Large number of legacy, i.e., non-WAP, phones
- WAP’s uncertain future
- Lack of widespread WAP content (as of yet)
- SMS is suitable for meeting major market needs
- SMS can be used as a kind of push technology, meaning the user doesn’t have to request delivery of information — it can be automatically sent to her/him
Based on the projected demand for wireless services, wireless messaging and access to financial services appear to be predominant, dwarfing the need for browsing (WAP’s core strength).
To harness SMS to deliver information to a cell phone, we propose using HTML forms available from major cell phone service providers to send messages to their subscribers. This helps us keep the solution simple and practical.
Web form scraping
A Web form is an HTML Webpage that submits information (such as user name, password, and so forth) to the server. Web form scraping is the act of interacting with an HTML Web form via a computer program. Most cell phone providers have HTML forms on their Websites, from which SMS messages can be sent to subscribers. Our solution will use this feature to deliver wireless content such as stock quotes without actually being connected to the wireless network.
Practical Java solution
We will demonstrate an application that you can use to push Web content to users’ cell phones via SMS. Some common application scenarios are:
- Stock trade confirmations/stock quotes
- News
- User-notification service
Our two-part solution is composed of a Java servlet and an application that delivers stock quotes to the user’s cell phone using SMS. You can also use it to easily deliver other services. The application fetches the stock information from well-known Websites and sends it to the user’s cell phone. The user must first create a profile that details the stock symbols, frequency of updates, and other relevant information.
The application works as follows:
-
Using an HTML form, a Java servlet retrieves and stores the user’s profile information. That information includes the following:
- Unique ID — email address, in this case
- Cell phone provider
- Cell phone number
- Frequency of updates
- Content preference — stock symbols, in this case
- A local text file stores the profile.
- A console application, which might run in a separate JVM, takes care of the timing and dispatch. It retrieves the stock quotes from Yahoo Quotes and determines which users should be sent messages, based on their frequency preferences.
- For every eligible user, the application passes the stock quotes to a handler, based on the user’s cell phone provider.
- The handler posts the data to an HTML form on the provider’s Website, which offers a Web-to-SMS interface.
- The application sleeps for an hour and returns to step 3.
The figure below illustrates the high-level flow. First, the user enters the profile via a desktop-based browser that communicates with the CellQuotes
servlet. That servlet stores the profile in a simple flat file, which is then read by the TimeWorker
application. Based on the profile settings, the TimeWorker
application retrieves the stock quotes from a datastream provider, e.g., and posts to the appropriate cell provider’s HTML form. Then the data forwards to the appropriate cell phone via SMS.

Solution architecture
Our solution uses the following classes:
(Note: you can download the entire source code from Resources.)
Profile.java
encapsulates user-specific informationProfileReader.java
reads profiles from the flat file asProfile
objectsProfileWriter.java
writesProfile
objects to the flat fileConstants.java
contains constants such as profile filenameCellQuotes.java
is the servlet that provides the Web interface to our solutionTimeWorker.java
is the behind-the-scenes application that takes care of actual dispatchCellProvider.java
is the interface that must be extended in order to add cellular service providersCellProviderSelector.java
is the factory that returns the appropriateCellProvider
implementation
The other classes are merely helpers, so we’ll concentrate on the CellQuotes
servlet and the TimeWorker
application, which share a common resource: the transaction file. The servlet writes profiles to the transaction file; the application reads new profiles from the file and adds them to the main store. Therefore, the transaction file needs to be synchronized. In a simplistic approach, we have designated a token lockFile
. If the servlet finds that the lockFile
exists, then it assumes the application is reading from the transaction file and waits its turn. If the applicationfinds that the lockFile
exists, then it assumes the servlet is writing to the transaction file and waits its turn. We have also provided a time-out functionality to avoid resource starvation and deadlock.
The code for the CellQuotes
servlet is as follows:
public class CellQuotes extends HttpServlet
{
public void doGet(...) throws ServletException
{
// return HTML Form to user
// We do this by simply reading a local file and dumping it to the response stream
}
public void doPost(...) throws ServletException
{
// extract profile information from POST
// assume its already been validated by JavaScript on client
// save parameters as new Profile object
...
// Write the profile to disk
writeProfile(profile);
...
// clean up
...
}
private void writeProfile(Profile p)
{
// synchronize on some static object, so that multiple servlet instances don't corrupt profiles file
synchronized(obj)
{
// check for existence of LockFile
if(Constants.lockFile.exists())
{
// waiting and timeout code goes here
}
// Since access is available, create lockfile and write to transaction file
ProfileWriter pw = new ProfileWriter(Constants.transactionFile);
prw.writeProfile(p);
...
}
}
}
You might wonder why we completed synchronization in such a roundabout manner, using lockFile
s, timeouts, and whatnot. Why not just synchronize on a common static object? Unfortunately, it’s not that simple. Our approach accounts for the fact that the servlet and application might run in different JVMs (which is almost a given); that means a common object cannot be established. (If you know of a better way to complete the synchronization, please let us know.)
The CellQuotes
servlet stores the user’s profile in a flat file. The TimeWorker
application adds that profile to its main profile store and also acts on the stored profiles.
The TimeWorker
application must wake every hour to determine which users need to receive stock quotes, and to update its main profile store. It might have to send quotes not only to users with hourly frequencies, but also to those with 3-hour frequencies, 6-hour frequencies, and so on. How does the TimeWorker
decide which users to send to?
We have a counter representing the number of hours in a day (24). If the current hour is exactly divisible by the user’s set frequency, then that user must receive the quotes. Here’s an example.
Suppose we start the application at 00:00 hours and we have three users:
- A: requests that stocks be sent once every hour
- B: requests that stocks be sent once every 3 hours
- C: requests that stocks be sent once every 6 hours
The logic used by the TimeWorker
application to select eligible users every hour is illustrated below:
- At 00:00 hours, the counter equals 0. Zero is divisible by all three frequencies, so
TimeWorker
sends all users their quotes. - At 01:00 hours, the counter equals 1. Only user A receives quotes.
- At 02:00 hours, the counter equals 2. Again, only user A gets quotes.
- At 03:00 hours, the counter equals 3. Users A and B receive quotes.
- At 06:00 hours, the counter equals 6. All three users receive quotes.
Since each provider has its own Web interface for sending SMS messages, TimeWorker
must next send the quotes to each user’s cellular service provider. We accomplish that by using a Factory-style design pattern. For every cellular provider, there is an implementation of the CellProvider
interface. The list of those implementing classes is maintained in a text file. The CellProviderSelector
class has a getProvider(String ID)
method that determines the appropriate handler, based on the ID. It does so by using the Reflection API. Thus, adding new cellular providers to our solution is as easy as:
- Writing the implementation of the
CellProvider
interface and adding it to the classpath - Adding
Classname
to theCellProviderRegistry
text file - Hoping the whole contraption actually works
The last point is just a jibe at ourselves; of course it’ll work!
The following code shows the internals of the TimeWorker
application:
public class TimeWorker
{
private static int counter = 0;
public static void main(String argv[])
{
// we want the application to run continuously, so enter an infinite loop
while(true)
{
// check if Transaction file exists
// if yes, then new profiles must be merged with main store
if(Constants.transactionFile.exists())
{
// check if lockfile exists
if(Constants.lockFile.exists())
{
// waiting and timeout code goes here
...
}
// We have access to transaction file, so create lockfile and merge profiles
...
}
// Main store is up-to-date
while(...) // loop to read all user profiles
{
// read next profile
// check if user is "eligible" to be sent quotes
if(...)
{
// get quotes for user's stock symbols from quote.yahoo.com
// get CellProvider implementation from CellProviderSelector factory
CellProvider prov = CellProviderSelector.getCellProvider(profile.getProviderID());
// send quotes to this handler, which then sends SMS message
}
}
// All user-profiles have been dealt with, sleep now
counter++;
try
{
// sleep for an hour
Thread.sleep(1000*60*60);
}
catch(InterruptedException ie)
{
...
}
}
}
}
Limitations
Our techniques are quite simplistic and not performance-oriented — we would love to learn about any alternative approaches you have found. Our approach has some limitations. For instance, our solution is tightly coupled with Web forms. Since Web form scraping is used as a means for sending SMS messages, the implementation of CellProvider
can easily become outdated.
We also suggest some areas of possible improvement:
- You can make provisions to allot user accounts, where users can edit/change their preferences.
- For a truly complete application, you can add a dial-in component using Java Telephony API. That will allow users to simply call a number to enable/disable the service.
- User profiles and cell provider information can be stored in XML files.
Conclusion
Our solution uses a Java servlet and application combination to illustrate an easy way to provide value-added services to the large number of people who have the ability to receive text messages (SMS-enabled) on their cell phones. Until the clouds surrounding WAP’s future clear and a better protocol arrives to enrich mobile users’ experience, SMS can be a viable alternative. SMS’s capability to serve applications is limited only by one’s imagination and ingenuity.