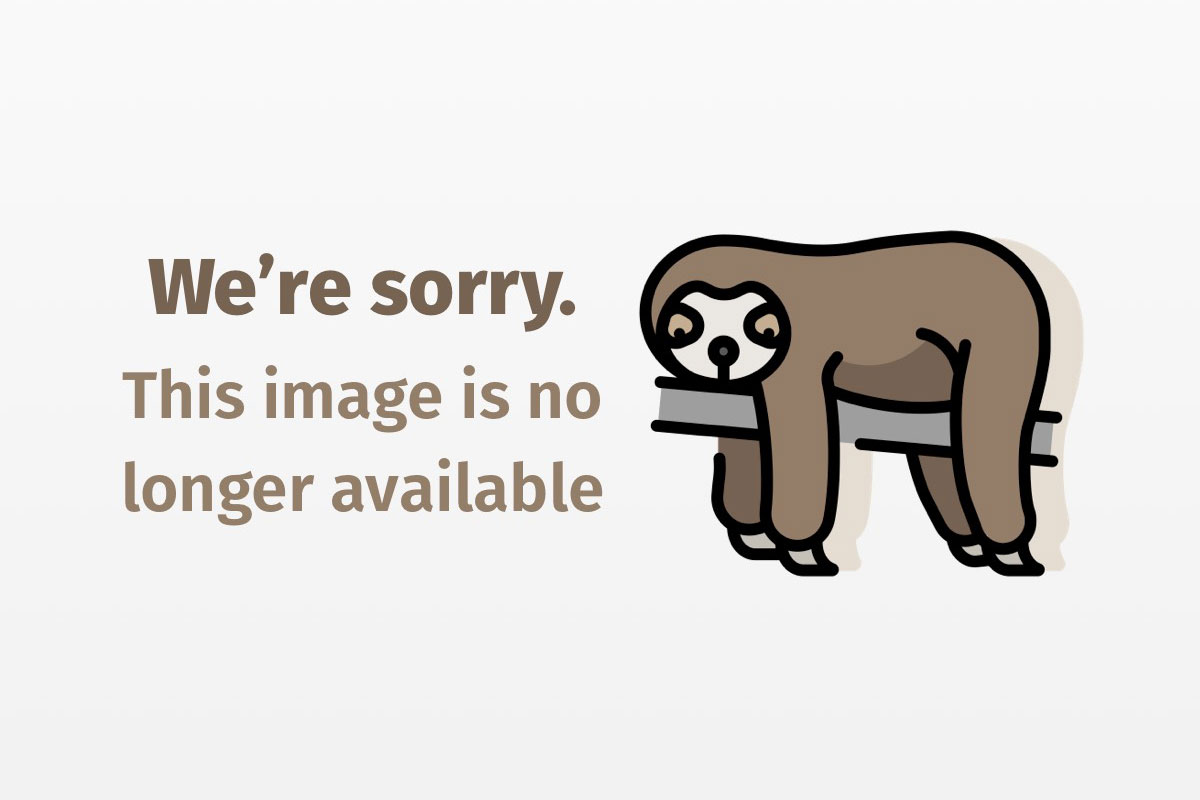
Two if by sea
Answers for LinkedList and outer/inner class questions
October 19, 2001
In this installment of Java Q&A, we’ll visit two quick but important questions. The first covers linked lists in Java, while the second looks at how to get an instance of an outer class inside the method of an inner class.
Q: I want to know about linked lists in Java. If the node is unconnected, does Java automatically delete it? Because in C, you still have to free the node. Or are there linked lists in Java?
A: If you implement linked lists correctly in Java, the node should get garbage collected after you completely disconnect it from the list. Just like other objects, if nothing refers to the object, the object will eventually get garbage collected. (For more information on garbage collection and application performance, see the excellent “Java Performance Programming, Part 1: Smart Object-Management Saves the Day.”)
Finally, you’ll be happy to learn that as of Java 2, Java includes a LinkedList
class.
Assume you have a class called OuterClass
with a private int variable named someValue
. Furthermore, assume OuterClass
has an inner class named InnerClass
.
Here’s the definition:
public class OuterClass
{
private int someValue;
private class InnerClass
{
public void someMethod()
{
int outerValue = OuterClass.this.someValue;
}
}
}
Take special note of InnerClass
‘s someMethod()
method. someMethod()
accesses OuterClass
‘s someValue
. In order to access the outer class instance from within an inner class, simply qualify the this
reference with the outer class’s name. In this case, the inner class can get access to the OuterClass
instance by employing OuterClass.this
.